2022. 7. 17. 17:12ㆍ유니티 unity
버전은 2022.2 기준입니다.
https://docs.unity3d.com/2022.2/Documentation/ScriptReference/Mathf.html
Mathf.Deg2Rad // public static float Deg2Rad;
(PI * 2) / 360, PI/180. 를 의미합니다. 보통 디그리(60분법)에다가 곱해서 라디안(효도법)을 구할 때 씁니다.
Mathf.Epsilon // public static float Epsilon;
작은 부동 소수점 값을 가져옵니다. 즉 0에 가까운 소수점을 반환합니다.
1.0f == (10.f/10.f) 이 값은 늘 true로 반환하지 않습니다.
두 값을 확인할 때는 Mathf.Epsilon를 활용해서 구할 수 있습니다.
bool isEqual(float a, float b)
{
if (a >= b - Mathf.Epsilon && a <= b + Mathf.Epsilon)
{
return true;
}
else
{
return false;
}
}
Mathf.Infinity // public static float Infinity;
양의 무한대
Mathf.NegativeInfinity // public static float NegativeInfinity;
음의 무한대
Mathf.PI // public static float PI;
파이 PI입니다. 3.14159265358979.......
Mathf.Rad2Deg // public static float Rad2Deg;
라디안을 디그리값으로 변환해주는 함수입니다. 360 / (PI * 2) 입니다.
1라디안은 57.29578도와 같습니다.
---------------------
메소드함수
Mathf.Abs // public static float Abs(float f);
절대값을 반환합니다.
Mathf.Abs(-3)이 값은 3 입니다.
Mathf.Acos // public static float Acos(float f);
arc-cosine를 반환합니다. 역코사인으로 라디안을 반환합니다
Acos를 이용하면 두 벡터의 각도를 알 수 있습니다.
내적을 구하는 공식은
벡터가 A 와 B가 있다면
Vector3.Dot(A, B) = Vector3.Magnitude(A) * Vector3.Magnitude(B) * Mathf.Cos(θ);
↓
Mathf.Cos(θ) = Vector3.Dot(A, B) / Vector3.Magnitude(A) / Vector3.Magnitude(B);
↓
θ = Mathf.ACos( Vector3.Dot(A, B) / Vector3.Magnitude(A) / Vector3.Magnitude(B) );
예시로 플레이어 시야 각안에 들어왔는지 확인하는 함수
float Angle = 70f; //시야각 70도로 설정
Vector3 targetDir = (target.transform.position - player.transform.position).normalized;
//방향을 구하고.
float dot = Vector3.Dot(player.transform.forward, targetDir);
//Dot함수를 이용해 두 벡터의 내적을 구한다.
//float theta = Mathf.Acos(dot/Vector3.Magintude(player.tranform.forward)/Vector3.Magintude(targetDir)) * Mathf.Rad2Deg;
// 라디안이기때문에 각도를 구할려면 Rad2Deg를 곱해준다
// Vector3.Magintude(player.tranform.forward)/Vector3.Magintude(targetDir) 둘다 노멀벡터이기때문에 | 1 || 1 | = 1 이다
float theta = Mathf.Acos(dot) * Mathf.Rad2Deg; // 이렇게 사용
//내적으로 구하기때문에 0~180도 사이의 값이 나온다.
if (Angle<=theta)
{
Debug.Log("시야각 70도안에 들어왔습니다.");
}
Mathf.Approximately // public static bool Approximately(float a, float b);
위에 Mathf.Epsilon를 활용한 함수입니다 bool 값을 반환합니다.
if (Mathf.Approximately(1.0f, 10.0f / 10.0f))
{
Debug.Log("이 값은 같습니다.");
}
Mathf.Asin // public static float Asin(float f);
arc-sin를 반환합니다. 역sin , 라디안을 반환합니다
Mathf.Atan // public static float Atan(float f);
acs-tangent를 반환합니다. 역 tan , 라디안을 반환합니다.
각도를 구할 때 사용합니다
예시 Mathf.Atan(y의길이 / x의길이)
Mathf.Atan2 // public static float Atan2(float y, float x);
acs-tangent를 반환합니다. 역 tan , 라디안을 반환합니다.
위에 있는 Atan랑 Atan2가 있는데 둘 다 똑같은데 뭐가 다르냐면
Atan는 y/x 를 하는 과정에서 둘 중 하나가 0이라면 오류가 발생하기 때문에
Atan2에서 인자를 두 개 받아서 계산하기 때문에 오류가 발생을 안 한다.
보통 Atan2를 쓴다.
Mathf.Ceil // public static float Ceil(float f);
크거나 같은 가장 작은 정수값을 반환합니다.(올림)
// 결과 10
Debug.Log(Mathf.Ceil(10.0F));
// 결과 11
Debug.Log(Mathf.Ceil(10.2F));
// 결과 11
Debug.Log(Mathf.Ceil(10.7F));
// 결과 -10
Debug.Log(Mathf.Ceil(-10.0F));
// 결과 -10
Debug.Log(Mathf.Ceil(-10.2F));
// 결과 -10
Debug.Log(Mathf.Ceil(-10.7F));
Mathf.CeilToInt // public static int CeilToInt(float f);
크거나 같은 가장 작은 정수값을 Int형으로 반환합니다.(올림)
// 결과 10
Debug.Log(Mathf.CeilToInt(10.0f));
// 결과 11
Debug.Log(Mathf.CeilToInt(10.2f));
// 결과 11
Debug.Log(Mathf.CeilToInt(10.7f));
// 결과 -10
Debug.Log(Mathf.CeilToInt(-10.0f));
// 결과 -10
Debug.Log(Mathf.CeilToInt(-10.2f));
// 결과 -10
Debug.Log(Mathf.CeilToInt(-10.7f));
Mathf.Clamp// public static float Clamp(float value, float min, float max);
value 값을 최솟값~최댓값 사이로 고정합니다.
public static float Clamp(float value, float min, float max)
{
if ((double) value < (double) min)
value = min;
else if ((double) value > (double) max)
value = max;
return value;
}
예시 체력 설정
public float currentHp;
public float HpMax;
void Heal(float value)
{
currentHp += value;
currentHp = Mathf.Clamp(currentHp, 0, HpMax);
//체력은 0~최대체력값 사이로 설정됩니다.
}
Mathf.Clamp01// public static float Clamp01(float value);
value 0에서 1의 값을 돌려줍니다.
public static float Clamp01(float value)
{
if ((double) value < 0.0)
return 0.0f;
return (double) value > 1.0 ? 1f : value;
}
Mathf.ClosestPowerOfTwo// public static int ClosestPowerOfTwo(int value);
가장 가까운 2의 n 제곱 값을 반환
// 결과 8
Debug.Log(Mathf.ClosestPowerOfTwo(7));
// 결과 16
Debug.Log(Mathf.ClosestPowerOfTwo(19));
Mathf.CorrelatedColorTemperatureToRGB// public static Color CorrelatedColorTemperatureToRGB(float kelvin);
켈빈(절대온도)을 RGB 색상으로 바꿔줍니다.
켈빈은 1000 ~ 40000도 사이이어야 합니다.
Mathf.Cos// public static float Cos(float f);
Cos함수입니다. f값은 라디안을 넣어야 합니다.
허용 가능한 값은 -9223372036854775295 to 9223372036854775295 입니다.
public int numberOfSides;
public float polygonRadius;
public Vector2 polygonCenter;
void Update()
{
DebugDrawPolygon(polygonCenter, polygonRadius, numberOfSides);
}
// 다각형 그리는 함수
void DebugDrawPolygon(Vector3 center, float radius, int numSides)
{
// 코너 설정
Vector3 startCorner = new Vector3(radius, 0) + center;
// 코너를 맨처음으로 설정
Vector3 previousCorner = startCorner;
// 그리기 시작
for (int i = 1; i < numSides; i++)
{
// 계산
float cornerAngle = 2f * Mathf.PI / (float)numSides * i;
// 코너 설정
Vector3 currentCorner = new Vector3(Mathf.Cos(cornerAngle) * radius,0, Mathf.Sin(cornerAngle) * radius) + center;
// 전 코너와 현재 코너를 그려줍니다.
Debug.DrawLine(currentCorner, previousCorner);
// 이전 코너를 설정
previousCorner = currentCorner;
}
// 마지막 연결
Debug.DrawLine(startCorner, previousCorner);
}
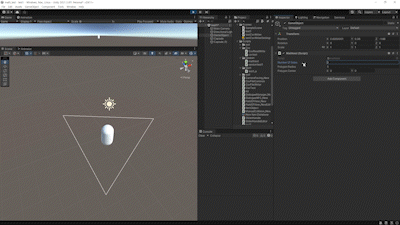
Mathf.DeltaAngle// public static float DeltaAngle(float current, float target);
두 각도의 최소 차이를 계산합니다.
public static float DeltaAngle(float current, float target)
{
float num = Mathf.Repeat(target - current, 360f);
if ((double) num > 180.0)
num -= 360f;
return num;
}
// 결과 90
Debug.Log(Mathf.DeltaAngle(1080, 90));
// 결과 10
Debug.Log(Mathf.DeltaAngle(80, 90));
// 결과 80
Debug.Log(Mathf.DeltaAngle(370, 90));
// 0
Debug.Log(Mathf.DeltaAngle(450, 90));
// -20
Debug.Log(Mathf.DeltaAngle(470, 90));
// 20
Debug.Log(Mathf.DeltaAngle(430, 90));
Mathf.Exp// public static float Exp(float power);
지정된 거급제곱으로 거듭제곱한 값을 반환합니다.
Mathf.FloatToHalf// public static ushort FloatToHalf(float val);
부동 소수점 값을 16비트 표현으로 인코딩합니다.
Mathf.Floor//public static float Floor(float f);
설정한 값 보다 작거나 같은 가장 큰 정수 값을 반환합니다(내림)
Mathf.FloorToInt// public static int FloorToInt(float f);
설정한 값 보다 작거나 같은 가장 큰 정수값을 int 형으로 반환합니다(내림)
Mathf.GammaToLinearSpace// public static float GammaToLinearSpace(float value);
감마에서 선형 색상 공간으로 값을 변환합니다.
Mathf.HalfToFloat// public static float HalfToFloat(ushort val);
반 정밀도 부동 소수점을 32비트 부동 소수점 값으로 변환합니다.
Mathf.Lerp//public static float Lerp(float a, float b, float t);
선형보간입니다
a, b값이 주어졌을 때 그 사이에 위치한 값을 추정하기 위하여 직선거리에 따라 선형적으로 계산하는 방법
t는 0~1 사이의 값을 줘야 합니다
예시
Lerp(0,10,0.5) = 5
Lerp(0,10,0.2) = 2
Lerp(0,10,0.4) = 4
Lerp(0,10,0.8) = 8
Lerp(0,10,1) = 10
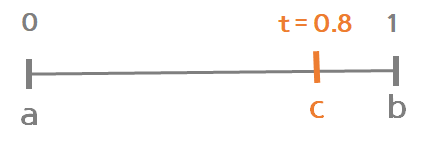
public static float Lerp(float a, float b, float t) => a + (b - a) * Mathf.Clamp01(t);
Mathf.InverseLerp//public static float InverseLerp(float a, float b, float value);
Lerp의 역함수입니다 0~1 값을 반환합니다.
Mathf.IsPowerOfTwo// public static bool IsPowerOfTwo(int value);
bool값을 반환하고 2의 거듭제곱일 때,true를 반환합니다.
// 결과 false
Debug.Log(Mathf.IsPowerOfTwo(7));
// 결과 true
Debug.Log(Mathf.IsPowerOfTwo(32));
Mathf.LerpAngle// public static float LerpAngle(float a, float b, float t);
Lerp 와 동일 하지만 각도 버전입니다.
시작 값과 끝 값이 0~360도 사이에 있어야 합니다.
보통 부드러운 회전을 구현할 때 씁니다.
public static float LerpAngle(float a, float b, float t)
{
float num = Mathf.Repeat(b - a, 360f);
if ((double) num > 180.0)
num -= 360f;
return a + num * Mathf.Clamp01(t);
}
Mathf.LerpUnclamped// public static float LerpUnclamped(float a, float b, float t);
Lerp와 동일하지만 t가 1을 넘어가도 그 이상을 값을 줍니다.
LerpUnclamped와 Lerp의 차이는 Lerp는 t값이 1이 넘어가면 최대 1로 설정이 되지만
lerpUmclamped는 그렇지 않습니다.
//결과 10
Debug.Log(Mathf.Lerp(0,10,1.5f));
//결과 15
Debug.Log(Mathf.LerpUnclamped(0,10,1.5f));
public static float Lerp(float a, float b, float t)
{
a + (b - a) * Mathf.Clamp01(t);
}
public static float LerpUnclamped(float a, float b, float t)
{
a + (b - a) * t;
}
Mathf.LinearToGammaSpace// public static float LinearToGammaSpace(float value);
주어진 값을 선형에서 감마(sRGB) 색 공간으로 변환합니다.
Mathf.Log// public static float Log(float f, float p);
f = 진수
p=base
log밑(base)진수
지정된 밑에서 지정된 숫자의 로그를 반환합니다.
// 결과 2.584963
Debug.Log(Mathf.Log(6, 2));
// 결과 2
Debug.Log(Mathf.Log(4, 2));
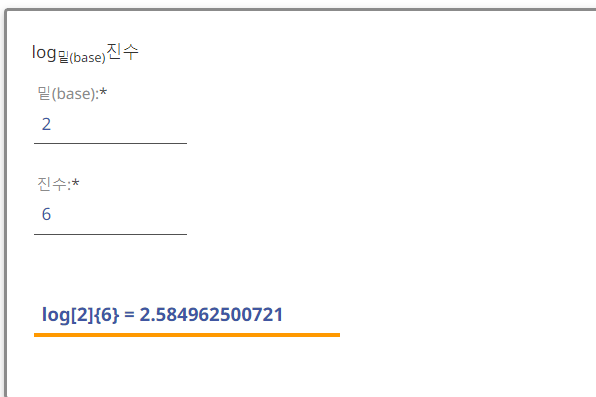
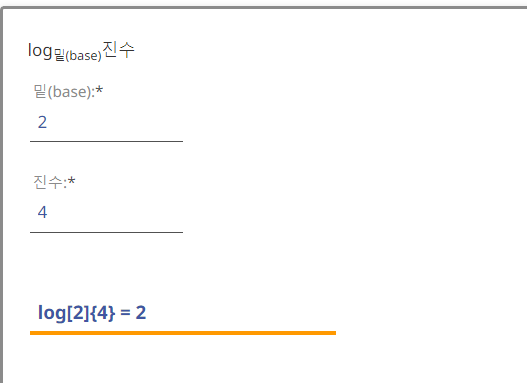
Mathf.Log10// public static float Log10(float f);
베이스가 10인 지정된 수의 로그를 반환합니다.
// 결과 2
Debug.Log(Mathf.Log10(100));
Mathf.Max// public static float Max(float a, float b);
a와 b 값 중 최대 값을 반환합니다
// 결과 2.4
Debug.Log(Mathf.Max(1.2f, 2.4f));
Mathf.Min// public static float Min(float a, float b);
a 와 b 값중 최소 값을 반환합니다.
// 결과 1.2
Debug.Log(Mathf.Min(1.2f, 2.4f));
Mathf.MoveTowards// public static float MoveTowards(float current, float target, float maxDelta);
값 current에서 target으로 이동합니다.
Mathf.Lerp 와 동일 하지만 maxDelta 가 음수라면 뒤로 갑니다.
public static float MoveTowards(float current, float target, float maxDelta)
{
(double) Mathf.Abs(target - current) <= (double) maxDelta ? target : current + Mathf.Sign(target - current) * maxDelta;
}
예제
public float currStrength;
public float maxStrength;
public float recoveryRate;
void Update()
{
currStrength = Mathf.MoveTowards(currStrength, maxStrength, recoveryRate * Time.deltaTime);
}
Mathf.MoveTowardsAngle// public static float MoveTowardsAngle(float current, float target, float maxDelta);
MoveTowards와 기능은 똑같지만 360도 돌 때, 정확하게 보간 되도록 합니다.
public static float MoveTowardsAngle(float current, float target, float maxDelta)
{
float num = Mathf.DeltaAngle(current, target);
if (-(double) maxDelta < (double) num && (double) num < (double) maxDelta)
return target;
target = current + num;
return Mathf.MoveTowards(current, target, maxDelta);
}
Mathf.NextPowerOfTwo// public static int NextPowerOfTwo(int value);
인수와 같거나 더 큰 2의 거듭제곱을 반환합니다.
//결과 8
Debug.Log(Mathf.NextPowerOfTwo(7));
//결과 256
Debug.Log(Mathf.NextPowerOfTwo(139));
//결과 256
Debug.Log(Mathf.NextPowerOfTwo(256));
Mathf.PerlinNoise// public static float PerlinNoise(float x, float y);
2d 평면 노이즈를 제작합니다.
반환 값은 0.0 ~ 1.0 사이입니다. 0보다 약간 작거나 1 보다 약간 클 수도 있습니다
Mathf.PingPong// public static float PingPong(float t, float length);
말 그대로 핑퐁 t값에서 length로 도달하면 다시 t값으로 가고 t값으로 가면 다시 length 값으로 갑니다.
t값은 자체적으로 증가하는 값(Time.time 및 Time.unscaledTime) 이어야 합니다.
public static float PingPong(float t, float length)
{
t = Mathf.Repeat(t, length * 2f);
return length - Mathf.Abs(t - length);
}
예제
게임 오브젝트가 x의 위치를 0~5 사이를 반복적으로 이동합니다.
private float t = 0;
private float speed = 10;
void Update()
{
t += Time.deltaTime;
transform.position = new Vector3(Mathf.PingPong(t*speed, 5), transform.position.y, transform.position.z);
}
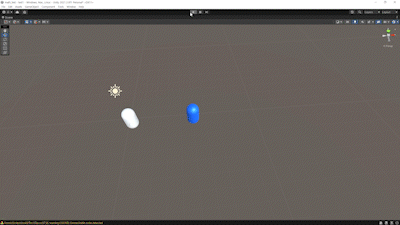
Mathf.Pow// public static float Pow(float f, float p);
거듭제곱을 반환합니다
f의 p 승
//결과 9
Debug.Log(Mathf.Pow(3,2));
//결과 27
Debug.Log(Mathf.Pow(3,3));
//결과 81
Debug.Log(Mathf.Pow(3,4));
Mathf.Repeat// public static float Repeat(float t, float length);
0~length 사이의 값을 반환합니다.
public static float Repeat(float t, float length)
{
Mathf.Clamp(t - Mathf.Floor(t / length) * length, 0.0f, length);
}
//결과 1
Debug.Log(Mathf.Repeat(11,10));
//결과 5
Debug.Log(Mathf.Repeat(5,10));
//결과 4
Debug.Log(Mathf.Repeat(14,10));
예제
게임오브젝트가 x의 위치를 0~5 사이를 이동합니다 5가 될 때 0으로 돌아갑니다
private float t = 0;
private float speed = 10;
void Update()
{
t += Time.deltaTime;
transform.position = new Vector3(Mathf.Repeat(t*speed, 5), transform.position.y, transform.position.z);
}
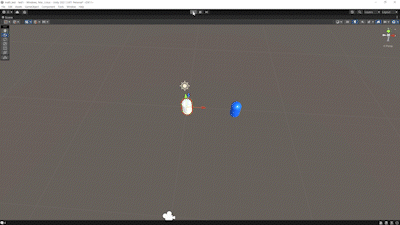
Mathf.Round// public static float Round(float f);
가장 가까운 정수를 반환합니다 (반올림)
// 결과 10
Debug.Log(Mathf.Round(10.0f));
// 결과 10
Debug.Log(Mathf.Round(10.2f));
// 결과 11
Debug.Log(Mathf.Round(10.7f));
// 결과 10
Debug.Log(Mathf.Round(10.5f));
// 결과 12
Debug.Log(Mathf.Round(11.5f));
// 결과 -10
Debug.Log(Mathf.Round(-10.0f));
// 결과 -10
Debug.Log(Mathf.Round(-10.2f));
// 결과 -11
Debug.Log(Mathf.Round(-10.7f));
// 결과 -10
Debug.Log(Mathf.Round(-10.5f));
// 결과 -12
Debug.Log(Mathf.Round(-11.5f));
Mathf.RoundToInt// public static int RoundToInt(float f);
Round와 같은데 int형으로 반환합니다.
Mathf.Sign// public static float Sign(float f);
양수일 때 1
음수일 때 -1 반환합니다.
//결과 -1
Debug.Log(Mathf.Sign(-10));
//결과 1
Debug.Log(Mathf.Sign(10));
Mathf.Sin// public static float Sin(float f);
sin 함수입니다. 라디안을 반환합니다.
예제는 위의 cos 함수 보시면 됩니다.
Mathf.SmoothDamp// public static float SmoothDamp(float current, float target, ref float currentVelocity, float smoothTime, float maxSpeed = Mathf.Infinity, float deltaTime = Time.deltaTime);
current에서 target으로 부드럽게 값이 변경됩니다.
currentVelocity = 현재 속도
smoothTime = 목표에 도달하는 데 걸리는 대략적인 시간, 작으면 작을수록 빠르게 도달합니다.
maxSpeed = 속도 최대치
public static float SmoothDamp(
float current,
float target,
ref float currentVelocity,
float smoothTime)
{
float deltaTime = Time.deltaTime;
float maxSpeed = float.PositiveInfinity;
return Mathf.SmoothDamp(current, target, ref currentVelocity, smoothTime, maxSpeed, deltaTime);
}
예제
0.3초 동안 해당타켓의 x값으로 부드럽게 이동하는 예제
public Transform target;
float smoothTime = 0.3f;
float yVelocity = 0f;
void Update()
{
float newPosition = Mathf.SmoothDamp(transform.position.x, target.position.x, ref yVelocity, smoothTime);
transform.position = new Vector3(newPosition, transform.position.y, transform.position.z);
}
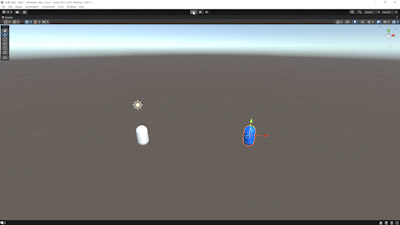
Mathf.SmoothDampAngle// public static float SmoothDampAngle(float current, float target, ref float currentVelocity, float smoothTime, float maxSpeed = Mathf.Infinity, float deltaTime = Time.deltaTime);
SmoothDamp와 똑같지만 각도 변화를 부드럽게 변화시켜주는 것
0도에서 300도로 이동하게 되면 0도에서 300도로 이동하는 게 아니고 0도에서 -60도로 가까운 방향으로 변환된다.
Mathf.SmoothStep// public static float SmoothStep(float from, float to, float t);
from to 사이의 보간 값을 반환해줍니다.
Lerp와 유사한 방식으로 보간합니다 그러나
Smooth는 부드럽게 이동하기 때문에 처음부터 점차적으로 속도가 빨라지고 끝으로 갈수록 느려집니다.
Mathf.Sqrt// public static float Sqrt(float f);
f의 제곱근을 반환합니다.
// 결과 2
Debug.Log(Mathf.Sqrt(4));
// 결과 3
Debug.Log(Mathf.Sqrt(9));
Mathf.Tan// public static float Tan(float f);
탄젠트 함수입니다. 라디안을 반환합니다.
'유니티 unity' 카테고리의 다른 글
unity ToolKit 와 UI Builder 이용해서 커스텀에디터만들기-1 (0) | 2022.07.20 |
---|---|
Unity로 node.js WebSocket 통신하기 -1 (0) | 2022.07.19 |
랜덤 원형안에 오브젝트 생성시키기 (0) | 2022.07.17 |
3d 오브젝트 드래그로 이동시키기 (0) | 2022.07.15 |
Handles와DrawGizmos 활용해서 시야각만들기 (0) | 2022.07.12 |